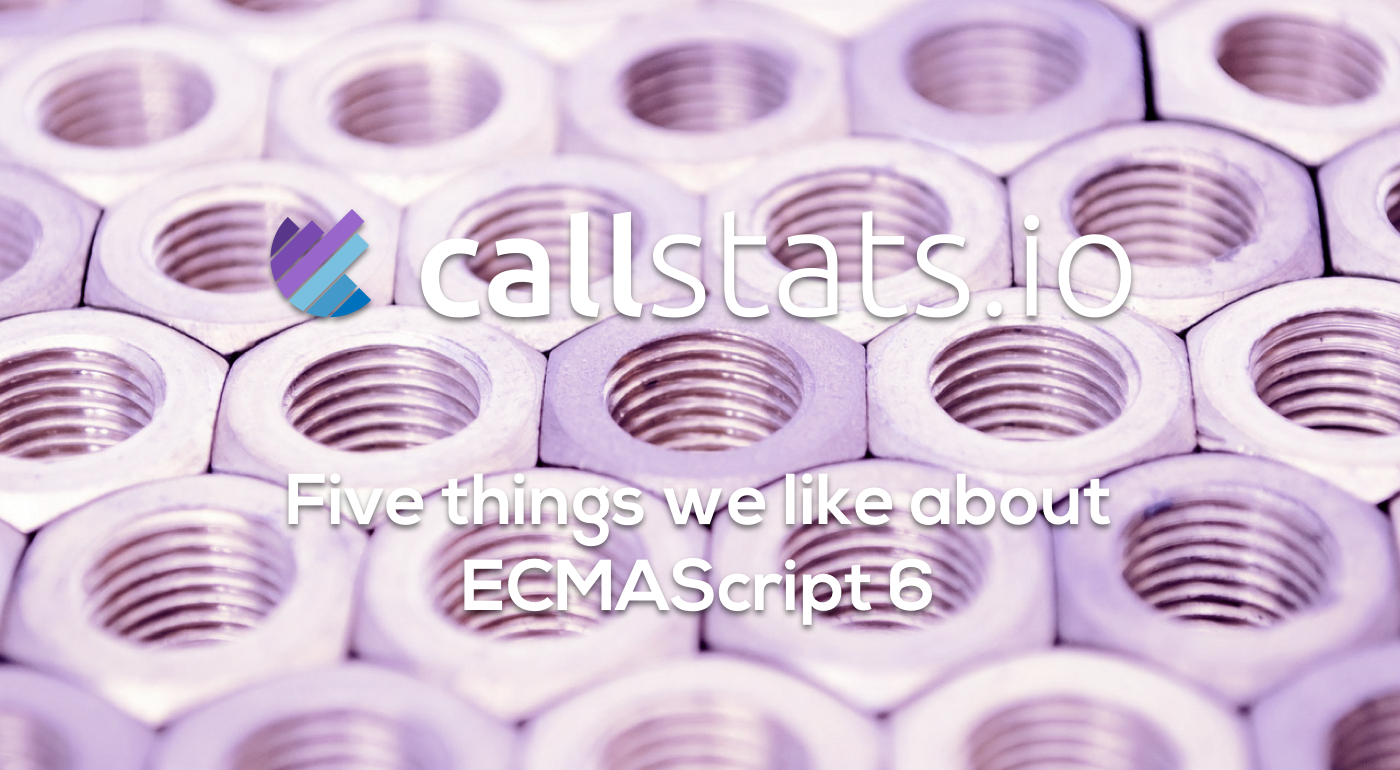
ECMAScript is a general purpose programming language. It is the basis for many technologies, such as, Javascript, Node.js and Microsoft’s Jscript. Although ECMAScript was initially based on Javascript, currently Javascript and Jscript offer features that are not defined in ECMAScript. Javascript is always evolving, mainly due to the vibrant developer community, which demands improvements and new features that already exist in some other language, for example, Python. This demand from the community lead to the ES5 proposal in 2011 and ES6 in 2015.
ES6 (ECMA2015) was released in mid-2015, the proposed changes were considered a major step for the Javascript language history. A full list of new features with codes are available at es6-features.org. Backward compatibility of ES6 with older versions of browsers is an issue, ergo products have to be careful about using the new features. You do not want customers complaining about the service that worked perfectly a week before. The status of ES6 adaption of browsers is available at kangax.github.io/compat-table/es6/. Voila, we have transpilers to save the day! Two commonly used transpilers are Babel and Traceur.
ES6 is great! In our experience, the following features of ES6 stand out when compared to ES5.
1. Classes
Classes are a fundamental feature of object-oriented programming and a class is expected to serve just one purpose. An object instantiates a class and the application builds functionality via these objects. In ES5 objects are created either via a prototype definition or using a function definition. In ES6, the keyword ‘class’ is codesugar
because under the hood it is still a prototype. Nevertheless, classes make Javascript code easier to understand and maintain.
class OutboundMonitor extends Monitor {
constructor(ssrc) {
super(ssrc);
//...
}
add(measurement) {
//...
super.add(measurement);
}
get throughput() {
return this.sentBytes / this.dtime
}
}
An example of classes in Javascript
2. Arrows
Map, reduce, and filter functions are commonly used for manipulating or aggregating values of a data structure. The caller iterates and performs an operation on each value over the collection. In-place definition of functions allows arbitrary operations on the collection. In ES5, the function keyword is used for creating a lambda function. Arrows are new in ES6, and indicate anonymous function definition. Additionally, arrows preserve the scope of this reference pointing to the caller object.
list1 = [1,2,3,4,5,6,7,8,9]
list2 = [4,6,7,2,5,7,6]
console.log(list1.reduce((r, v) => { return r + v; }) * list2.reduce((r, v) => { return r + v; }));
An example of arrows in Javascript
3. Maps
ES6 also introduced the map
type. Maps are associative data structures, in which elements are stored as a combination of key and value pairs. In previous versions of ES, objects were used as maps, but it made the object type overloaded. Prototype based programming allows to add attributes to objects dynamically. In this way objects can be used as maps, however iterating and performing operations on elements of the object needs to consider the own properties of the object. Instead, a map indicates more clearly what is the purpose of the variable, which is handy in code reviewing. WeakMaps are a subtype of maps, and they enable much more efficient caching and less probability for memory leaks.
m = new Map();
m.set('key1',1);
m.set('key2',2);
m.get('key1') === 1
for (let [ key, val ] of [...m].filter(([k,v]) => 1 < v).entries())
console.log(key + " = " + val);
An example of maps in Javascript
4. Default Parameter Values
It is not possible to overload a function in Javascript, although the number of the parameters passing to a method is arbitrary. A common solution in ES5 was to check whether a particular argument was undefined or not. In ES6, an argument may have default parameter in the case they are not specified (added after Chrome 49 and Firefox 15, but not supported in Safari yet).
function sum(a,b,c=5){
return a + b + c;
}
console.log(sum(1,2))
An example of default parameter values in Javascript
5. Promises
We left our favorite for the end! Promises and futures are basic tools for asynchronous execution in many programming languages. Asynchronous execution is performed by using setTimeout with some other combinations. Promises and futures are used for decoupling a calculation from the actual execution flow by making a promise for the calculation to be done, and the results are available later. Although the implementation of promises and futures is not yet perfect in Javascript, finally they can be used as native elements in ES6.
var authenticate = new Promise(function(resolve, reject) {
console.log("authenticate is in progess... asynchronously")
resolve(true);
});
console.log("Other things are executed")
authenticate.then((response) => {
if(response === true){
console.log("Authentication is successfully done")
}
});
An example of promises in Javascript
The latest version of the ECMAScript is documented at: www.ecma-international.org/ecma-262/7.0/index.html.
If you are interested in Javascript development, have at look at our careers page. We have many software development positions open!